AddScoped vs AddSingleton vs AddTransient:
Within the domain of ASP.NET Core, overseeing the creation and duration of objects, particularly concerning services, holds significant weight. Within the IServiceCollection interface, three distinct methodologies—AddScoped, AddTransient, and AddSingleton—govern the creation and dispersal of services throughout your application:
Transient Services
This methodology entails generating a fresh instance of the service with each request, ensuring a transient state. It proves most advantageous for lightweight services lacking persistent state
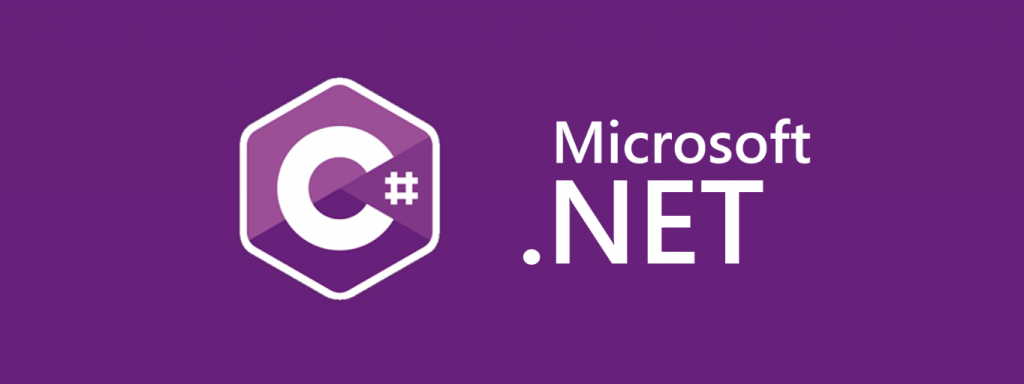
between requests.
Illustration:
In C#:
public class LoggingService
{
private readonly string _requestId;
public LoggingService(ILoggerFactory loggerFactory)
{
_requestId = Guid.NewGuid().ToString();
}
public void LogMessage(string message)
{
Console.WriteLine($"[{_requestId}] {message}");
}
}
services.AddTransient<LoggingService>();
Usage in a controller:
public class MyController
{
private readonly LoggingService _logger;
public MyController(LoggingService logger)
{
_logger = logger;
}
public void MyAction()
{
_logger.LogMessage("This is a new instance every time.");
}
}
This methodology guarantees that each invocation of MyAction results in a fresh LoggingService instance with a unique request ID.
Scoped Services
This method involves creating a single instance of the service for the duration of a designated request, typically synonymous with an HTTP request in ASP.NET Core. It is suitable for services requiring intra-request state maintenance while avoiding inter-request persistence.
Illustration:
In C#:
public class NotificationService
{
private readonly List<Message> _messages;
public NotificationService()
{
_messages = new List<Message>();
}
public void AddMessage(Message message)
{
_messages.Add(message);
}
public IEnumerable<Message> GetMessages()
{
return _messages;
}
}
services.AddScoped<NotificationService>();
Usage in a controller:
public class NotificationController
{
private readonly NotificationService _notifier;
public NotificationController(NotificationService notifier)
{
_notifier = notifier;
}
public void AddMessage(Message message)
{
_notifier.AddMessage(message);
}
public void DisplayMessages()
{
foreach (var msg in _notifier.GetMessages())
{
Console.WriteLine(msg.Content);
}
}
}
In this scenario, the NotificationService instance persists throughout a single request, facilitating consistent message management operations.
Singleton Services
This method spawns a sole instance of the service for the entire duration of the application’s lifecycle or application domain. Caution must be exercised, as improper state management may lead to unforeseen complications. It is most suitable for services with truly global scope, devoid of per-request isolation requirements.
Illustration:
In C#:
public class CacheService
{
private readonly Dictionary<string, object> _cache;
public CacheService()
{
_cache = new Dictionary<string, object>();
}
public void AddToCache(string key, object value)
{
_cache[key] = value;
}
public object GetFromCache(string key)
{
return _cache.ContainsKey(key) ? _cache[key] : null;
}
}
services.AddSingleton<CacheService>();
Usage in any controller or service:
public class CacheController
{
private readonly CacheService _cache;
public CacheController(CacheService cache)
{
_cache = cache;
}
public void AddToCache(string key, object value)
{
_cache.AddToCache(key, value);
}
public object GetFromCache(string key)
{
return _cache.GetFromCache(key);
}
}
This methodology ensures the persistence of a single CacheService instance throughout the application’s runtime.
Choosing the Suitable Service Lifetime
AddScoped vs AddSingleton vs AddTransient:
For lightweight, stateless services: Transient
For services requiring intra-request state persistence: Scoped
For globally applicable, application-wide services: Singleton (to be used judiciously)
By comprehending these service lifetimes, you can adeptly manage object instantiation and distribution within your ASP.NET Core applications, thereby ensuring a semblance of uniformity and predictability in their behavior.
AddScoped vs AddSingleton vs AddTransient
HTTP ERROR 500.30 – ASP.NET CORE APP FAILED TO START IN IIS [Solved]